Hare Krishna
he krishna karuna-sindho
dina-bandho jagat-pate
gopesa gopika-kanta
radha-kanta namostu te
O my dear Krishna, You are the friend of the distressed and the source of creation. You are the master of the gopis and the lover of Radharani. I offer my respectful obeisances unto You.
My Technical Certifications
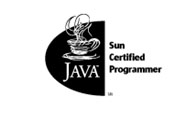
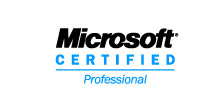

About Me
Mr. Sanjoy Roy has a Master Degree in Computer Management and a B.Sc. Degree in Electronics (with Computer Maintenance) from Pune University, India.
He is mostly involved in database application development. His responsibility includes system analysis, database design, front-end developments, proposal writing, training etc. He is also involved in developing web based Java applications for various clients like ROI Mailing System, Web Banking System and Intranet Mailing System for SSI (Software Solution Integrated, India) and Child Development Program âWorld Link (CDPWL-WEB) for FHI (Food for Hungry International,USA) etc.
Mr. Sanjoy is one of the early holder of SCJP (Sun Certified Java Programmer) from Aipath since January, 2002 and MCP(Microsoft Certified Professional) from Base since June 2002.
Mr. Sanjoy has received ICCR (Indian Council for Cultural Relationship) scholarship in 1996 for his education in India. Besides of his regular studies he has also completed first Level REIKI.
Computer Language: C, C++, Java, PHP, Oracle PL-SQL, Visual Basic, XML, FoxPro 2.5, Assembly. Databases : Oracle9i, MS-Access, SQL Server 2000, MySQL, PostgreSQL 7.3.2 Internet Tools Techniques: Applet, JDBC, JFC Swing, Servlets, Java Script, JSP, RMI, EJB, Java Beans, HTML, CSS, XML, XML-RPC, XSL
He is mostly involved in database application development. His responsibility includes system analysis, database design, front-end developments, proposal writing, training etc. He is also involved in developing web based Java applications for various clients like ROI Mailing System, Web Banking System and Intranet Mailing System for SSI (Software Solution Integrated, India) and Child Development Program âWorld Link (CDPWL-WEB) for FHI (Food for Hungry International,USA) etc.
Mr. Sanjoy is one of the early holder of SCJP (Sun Certified Java Programmer) from Aipath since January, 2002 and MCP(Microsoft Certified Professional) from Base since June 2002.
Mr. Sanjoy has received ICCR (Indian Council for Cultural Relationship) scholarship in 1996 for his education in India. Besides of his regular studies he has also completed first Level REIKI.
Computer Language: C, C++, Java, PHP, Oracle PL-SQL, Visual Basic, XML, FoxPro 2.5, Assembly. Databases : Oracle9i, MS-Access, SQL Server 2000, MySQL, PostgreSQL 7.3.2 Internet Tools Techniques: Applet, JDBC, JFC Swing, Servlets, Java Script, JSP, RMI, EJB, Java Beans, HTML, CSS, XML, XML-RPC, XSL
Recent Tags
.htaccess 7-Zip ActivePerl Add-Ons Adobe Flash Apache Australia Change Selective Area Checklist Citizenship Ceremony Cobian Backup Coloring Color Picker ColorZilla CPanel Creloaded Creloaded 6.2 Cross-Browser CSV Parser Daylight DebugBar Debugging Tool DEFLATE Directory e-commerce Evolved ExtJS Eyedropper Family Fiddler filter Firebug Firefox Flex Framework Freelance Freeware Gmail Good Day Google ladder Grameenphone ICCR Icon IE Indexing Internet Explorer Developer Toolbar JavaScript JavaScript/Flash library Job Joomla jQuery Lightbox Linux Linux Command Magento MySQL Opencart Open Source Opensource osCommerce Parser Password Photoshop PHP Plugin Product Session shell script Shopping Cart Theme Thunderbird URL Validation webalizer Webmail ClientRecent Posts
- Navman white or black screen fix
- Javascript Validate two input fields with the same name?
- Copy recursively directory structure using Linux Command
- Great JS Webtool for Developers: Aardvark
- Online ICON (*.ico) File Creator
- Free Canon DSLR Photgraphy Course
- jQuery UI icons
- Top Free Secure SFTP Clients for Windows
- Impressive WordPress Themes
- Opencart Removing Estimate Shipping & Taxes v1.5.1
Archives
M | T | W | T | F | S | S |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 | 31 |
Bangla Radio
āĻļā§āύā§āύ
āĻ
āĻĄāĻŋāĻ āĻ
āύā§āώā§āĻ āĻžāύ
|